Unleash the power of JavaScript with our comprehensive beginner’s guide. Learn the essential skills to become a coding pro!

Image courtesy of via DALL-E 3
Table of Contents
Introduction to JavaScript
Welcome, young programmers! Today, we’re diving into the exciting world of JavaScript, a programming language that is super important for beginners like you to learn. But first things first, what exactly is JavaScript?
What is JavaScript?
JavaScript is a programming language that is used to make websites interactive. Think of it as the magic ingredient that brings web pages to life! By writing JavaScript code, developers can create cool features like interactive buttons, animations, and games that you see on your favorite websites.
Why Learn JavaScript?
Learning JavaScript is essential for anyone interested in web development. It’s like learning a new language that helps you communicate with computers and tell them what to do. JavaScript is used in almost every website you visit, whether it’s a social media platform, an online game, or an educational site. So, by mastering JavaScript, you’ll be able to create your own awesome web projects and bring your ideas to life on the internet!
Setting Up Your Environment
Before we dive into the exciting world of JavaScript coding, we need to set up our environment. This means getting everything ready on your computer so you can start writing and testing your code. Don’t worry, it’s easier than it sounds! Let’s go through the steps together.
Choosing a Text Editor
A text editor is like a digital notebook where you write your code. For beginners, it’s best to use a simple and user-friendly text editor like Visual Studio Code or Sublime Text. These tools have features that make coding easier, such as color-coding and auto-completion.
Using Your Browser
Your browser, like Chrome or Firefox, is where you’ll see your JavaScript code come to life. Modern browsers have built-in tools that allow you to run and test your JavaScript code directly. You can even see the changes you make to your code in real-time!
Basic Setup Steps
Now, let’s walk through the basic setup steps:
- Download and install a text editor like Visual Studio Code or Sublime Text.
- Open your text editor and create a new file.
- Write a simple JavaScript program, like displaying a message on the screen.
- Save your file with a .js extension (e.g., mycode.js).
- Open your browser and create an HTML file to link to your JavaScript code using the
<script>
tag. - Run your HTML file in the browser and see your JavaScript code in action!
There you go! You’re now all set up and ready to start coding in JavaScript. In the next section, we’ll explore the basics of JavaScript syntax, variables, and data types.
JavaScript Basics
JavaScript is like a set of rules that you need to follow when you write code. These rules are called syntax. Think of it like following a recipe – if you don’t follow the steps correctly, your cookies might not turn out right! In JavaScript, you need to pay attention to things like where you put your semicolons (;) and braces ({ }) to make sure your code works the way you want it to.
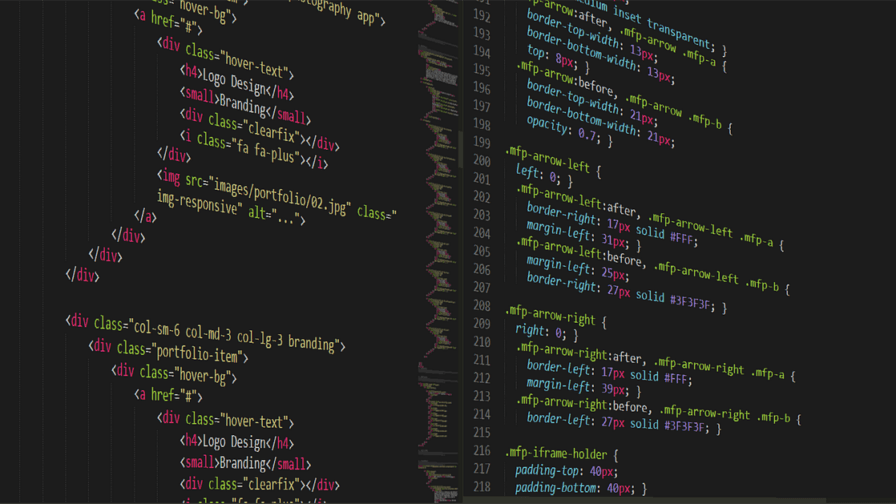
Image courtesy of www.codemotion.com via Google Images
Variables and Data Types
Variables in JavaScript are like containers that hold different types of information. You can store numbers, words, or even whole sentences in variables. For example, you could have a variable called ‘age’ that holds the number 11, or a variable called ‘name’ that holds the word ‘Sara’.
There are different types of data you can store in variables, like numbers (1, 2, 3), strings (words or sentences), and booleans (true or false). Each type of data is used for different things in JavaScript, so it’s important to know which type you need for your code to work correctly.
Working with Numbers and Strings
When coding in JavaScript, you’ll often find yourself working with numbers and strings. Understanding how to handle them is essential for building interactive websites. Let’s dive into the basics of working with numbers and strings in JavaScript!
Basic Math Operations
Numbers play a significant role in programming. JavaScript allows you to perform basic math operations like addition, subtraction, multiplication, and division. For example, if you want to add two numbers together, you can use the addition operator (+). Here’s an example:
let num1 = 5;
let num2 = 3;
let sum = num1 + num2; // sum will be 8
Manipulating Strings
Strings are sequences of characters, such as letters, numbers, or symbols. In JavaScript, you can manipulate strings by combining or extracting parts of them. One common operation is concatenation, which means joining two or more strings together. Here’s an example:
let greeting = 'Hello, ';
let name = 'John';
let message = greeting + name; // message will be 'Hello, John'
By understanding how to work with numbers and strings in JavaScript, you’ll be well on your way to creating dynamic and engaging web applications!
Introducing Functions
A function in JavaScript is like a recipe or a set of instructions that can be called upon to perform a specific task. It helps to keep your code organized, efficient, and reusable. To define a function, you start with the keyword “function,” followed by a name you choose for the function, then parentheses, and finally curly braces. Inside the curly braces, you write the code that you want the function to execute when it is called.
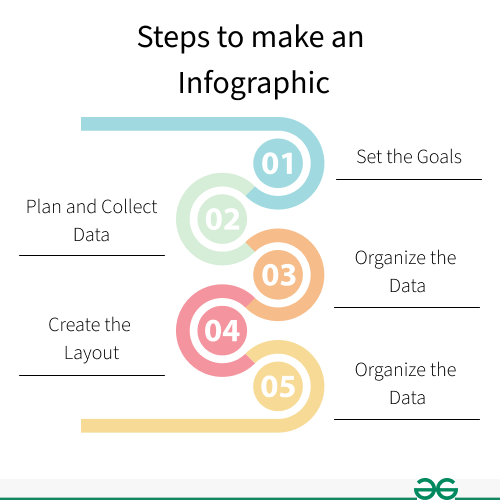
Image courtesy of www.geeksforgeeks.org via Google Images
Calling Functions
Once you have defined a function, you can call it by using its name followed by parentheses. This tells the computer to execute the code inside the function. Calling a function allows you to use the same set of instructions multiple times without having to rewrite them. It’s like using a magic spell whenever you need to perform a specific action in your code.
Conditional Statements
Conditional statements in JavaScript allow the code to make decisions based on certain conditions. One type of conditional statement is the ‘if’ statement. The ‘if’ statement checks a specified condition. If the condition is true, the code inside the ‘if’ block will run. If the condition is false, the code inside the ‘if’ block will be skipped.
Else and Else If
There are situations where you might want to consider multiple conditions in your code. This is where ‘else’ and ‘else if’ come into play. After an ‘if’ statement, you can use an ‘else’ statement to specify a block of code that will execute if the condition in the ‘if’ statement is false. Additionally, the ‘else if’ statement allows you to check another condition if the previous ‘if’ statement is false. This way, you can handle various scenarios within your code.
Loops in JavaScript
In JavaScript, a for loop is used to repeat a block of code a fixed number of times. It consists of three parts: initialization, condition, and increment/decrement. Let’s break it down:

Image courtesy of www.pinterest.com via Google Images
1. Initialization: This is where you set a starting point. For example, you can declare a variable and set it to 0.
2. Condition: Here, you specify the condition that must be true for the loop to continue. For instance, you can say the loop should run as long as the variable is less than 5.
3. Increment/Decrement: This is where you update the variable to move closer to the end condition. You can increase its value by 1 each time the loop runs.
Here’s an example of a for loop that counts from 0 to 4:
“`javascript
for (let i = 0; i < 5; i++) {
console.log(i);
}
“`
While Loop
A while loop in JavaScript repeats a block of code as long as a specified condition is true. It’s like saying “Keep doing this until something changes.” Here’s how it works:
1. You set an initial condition before entering the loop.
2. The block of code inside the loop runs as long as the condition is true.
3. You need to ensure the condition changes at some point to prevent an infinite loop.
Here’s an example of a while loop that counts from 0 to 4:
“`javascript
let i = 0;
while (i < 5) {
console.log(i);
i++;
}
“`
Understanding loops is crucial in programming as they help automate repetitive tasks and make your code more efficient. Practice using for and while loops in JavaScript to become a pro coder in no time!
JavaScript in Action
JavaScript can bring life to dull web pages by making them interactive. To include JavaScript code in an HTML file, all you need is the <script>
tag. This tag tells the browser to run the JavaScript code enclosed within it. It’s like giving your web page a brain to process and respond to user actions.
Simple Interactive Web Page
Let’s try a fun project to showcase how JavaScript can make a web page interactive. How about creating a game where a player can click on buttons to change the color of a shape? We can use JavaScript to listen for these button clicks and change the color accordingly. It’s like magic happening right on your screen!
Conclusion and Next Steps
Now that you’ve learned the basics of JavaScript, it’s time to recap what you’ve covered and think about what comes next on your programming journey.
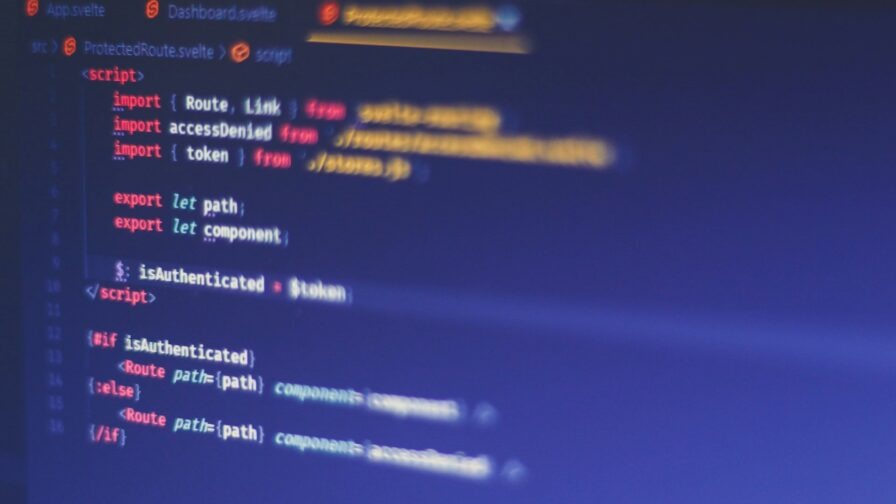
Image courtesy of www.codemotion.com via Google Images
Recap
In this guide, you’ve discovered what JavaScript is and why it’s an essential programming language for beginners. You’ve set up your coding environment, delved into JavaScript syntax, learned about variables, data types, numbers, and strings, explored functions, conditional statements, and loops, and seen how JavaScript can bring web pages to life.
Remember, learning JavaScript is a process that takes time and practice. Don’t be discouraged by challenges you may face along the way. Keep experimenting, building projects, and seeking help when needed.
Further Learning
If you’re eager to deepen your understanding of JavaScript, there are endless resources available to support your learning journey. Here are a few recommended websites, books, and tutorials to help you continue your exploration:
- Mozilla Developer Network – JavaScript Guide
- JavaScript.info – Modern JavaScript Tutorial
- “JavaScript: The Definitive Guide” by David Flanagan
These resources will help you expand your knowledge, tackle more complex concepts, and apply JavaScript in exciting new ways. Remember, practice makes perfect, so keep coding and exploring the endless possibilities of JavaScript!
Want to turn these SEO insights into real results? Seorocket is an all-in-one AI SEO solution that uses the power of AI to analyze your competition and craft high-ranking content.
Seorocket offers a suite of powerful tools, including a Keyword Researcher to find the most profitable keywords, an AI Writer to generate unique and Google-friendly content, and an Automatic Publisher to schedule and publish your content directly to your website. Plus, you’ll get real-time performance tracking so you can see exactly what’s working and make adjustments as needed.
Stop just reading about SEO – take action with Seorocket and skyrocket your search rankings today. Sign up for a free trial and see the difference Seorocket can make for your website!
Frequently Asked Questions (FAQs)
As you begin your journey to learn JavaScript, you might have some questions in mind. Here are some common queries that beginners often have:
What is JavaScript?
JavaScript is a programming language that is used to make websites interactive. It allows you to add special effects, manipulate content on the page, and respond to user actions.
Why Learn JavaScript?
Learning JavaScript is important because it is widely used in web development. Many websites and web applications rely on JavaScript to provide dynamic and engaging user experiences. By learning JavaScript, you can unlock the potential to create interactive websites and enhance your coding skills.
How can I get started with JavaScript?
To start learning JavaScript, you can follow the steps outlined in this guide. Begin by setting up your coding environment, understanding the basics of JavaScript syntax, working with variables and data types, and exploring functions, conditional statements, and loops. Practice writing code and building simple projects to reinforce your learning.
Is JavaScript difficult to learn?
While learning any new programming language can be challenging, JavaScript is a beginner-friendly language that is relatively easy to pick up. By breaking down concepts into manageable chunks, practicing regularly, and seeking help from online resources, tutorials, and communities, you can gradually build your proficiency in JavaScript.
What are some resources for further learning?
For those eager to delve deeper into JavaScript, there are numerous online resources available. Websites like Codecademy, W3Schools, and MDN Web Docs offer tutorials, exercises, and documentation to help you enhance your JavaScript skills. Additionally, books like “Eloquent JavaScript” by Marijn Haverbeke and “JavaScript: The Good Parts” by Douglas Crockford are highly recommended for aspiring JavaScript developers.
By exploring these resources, practicing regularly, and building fun projects, you can continue to grow as a JavaScript programmer and unlock new possibilities in web development.